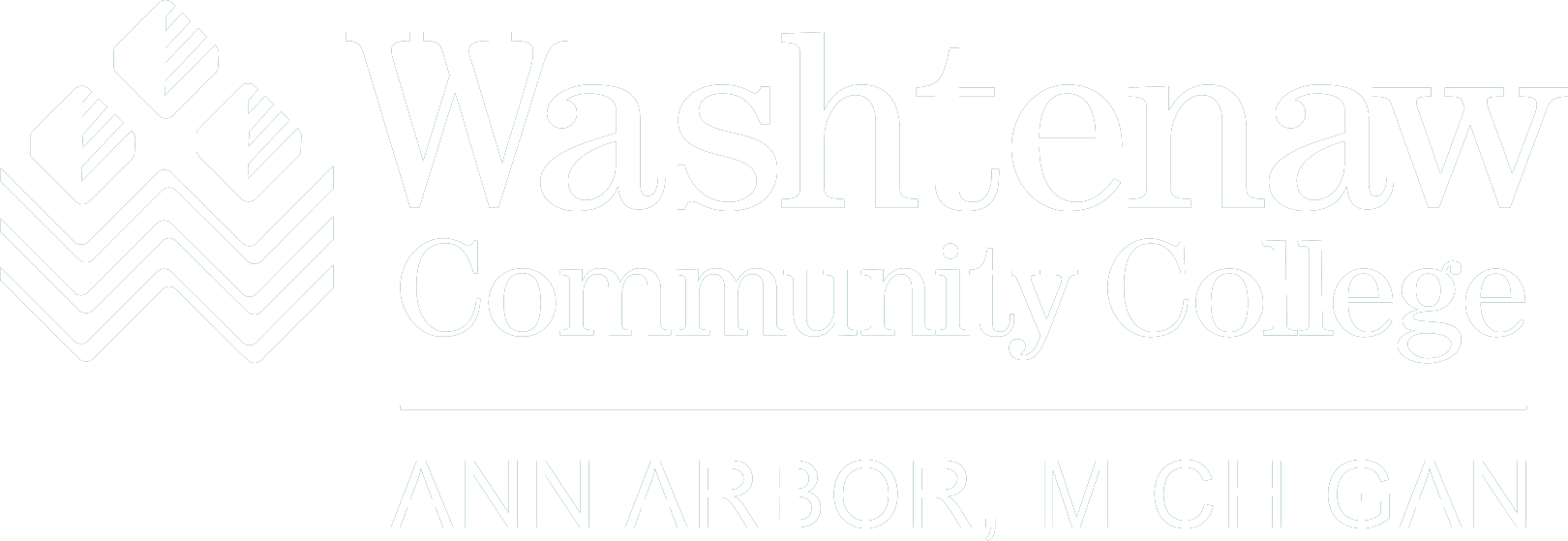
Linux Expansion
Think of Linux expansions like a magic wand that transforms your commands into something more powerful. Just like how a chef uses different tools to prepare ingredients, Linux gives you special characters and patterns that automatically expand into useful information. These expansions help you work faster and smarter with your files and commands.
Quick Reference
Expansion Type | What It Does | Common Use |
---|---|---|
Pathname | Matches files using patterns | Finding files, listing directories |
Tilde | Expands to home directories | Navigating to home folders |
Arithmetic | Performs math operations | Calculations in commands |
Brace | Creates multiple strings | Making similar files/folders |
Parameter | Uses variable values | Working with variables |
Command Substitution | Uses command output | Combining commands |
When to Use Expansions
- When you need to work with multiple files at once
- When you want to save typing time
- When you need to perform calculations
- When you want to create similar files/folders
- When you need to use command output in other commands
Pathname Expansion (Wildcards)
Think of pathname expansion like a search pattern for files. It's like telling your computer "find all files that match this pattern" instead of listing each file individually.
Pattern | What It Matches | Example |
---|---|---|
* |
Any number of characters | *.txt matches all text files |
? |
Exactly one character | file?.txt matches file1.txt, fileA.txt |
[abc] |
Any character in the set | [a-c]*.jpg matches a.jpg, b.jpg, c.jpg |
[!abc] |
Any character NOT in the set | [!0-9]*.txt matches files not starting with numbers |
[[:class:]] |
Character class (alnum, alpha, digit, etc.) | [[:digit:]]*.txt matches files starting with numbers |
{pattern1,pattern2} |
Multiple patterns | *.{txt,md} matches .txt and .md files |
** |
Recursive matching | **/*.txt matches .txt files in all subdirectories |
Practical Examples
# List all text files in current directory
ls *.txt
# Find files with single character names
ls file?.txt
# List all jpg files starting with a, b, or c
ls [a-c]*.jpg
# Find all files with numbers in their names
ls *[0-9]*
# Find files NOT starting with numbers
ls [!0-9]*
# Find files starting with letters
ls [[:alpha:]]*
# Find both .txt and .md files
ls *.{txt,md}
# Find all .txt files in all subdirectories
ls **/*.txt
# Find files with specific extensions
ls *.{jpg,png,gif}
# Find files with specific patterns
ls *[0-9][a-z].txt
Tilde Expansion
Think of the tilde (~) as a shortcut to your home directory. It's like having a "Home" button that takes you to your personal space on the computer.
Practical Examples
# Go to your home directory
cd ~
# List files in your Documents folder
ls ~/Documents
# Copy a file to your Downloads
cp file.txt ~/Downloads/
# Check another user's home directory
echo ~username
Arithmetic Expansion
Think of arithmetic expansion like a calculator built into your commands. It lets you do math right in your terminal!
Practical Examples
# Basic math operations
echo $((5 + 3)) # Addition
echo $((10 - 2)) # Subtraction
echo $((4 * 3)) # Multiplication
echo $((20 / 4)) # Division
# Using variables
count=5
echo $((count + 1))
# Complex calculations
echo $(( (5 + 3) * 2 )) # Parentheses work too!
Brace Expansion
Think of brace expansion like a copy machine that creates multiple versions of similar names. It's perfect for when you need to create several similar files or folders.
Practical Examples
# Create multiple files
touch file{1,2,3}.txt
# Create numbered folders
mkdir folder_{1..5}
# Create files with leading zeros
touch image_{001..005}.png
# Combine with other text
echo project_{alpha,beta,gamma}_test
Parameter Expansion
Think of parameter expansion as a way to use and modify variables in your commands. It's like having a toolbox for working with stored information.
Practical Examples
# Show your home directory
echo $HOME
# Show your username
echo $USER
# Show your PATH
echo ${PATH}
# Set default values
echo ${UNSET_VARIABLE:-default_value}
Command Substitution
Think of command substitution as a way to use the output of one command inside another command. It's like having a conversation where one command's answer becomes part of another command's question.
Practical Examples
# Get current date
echo "Today is: $(date)"
# Count files in directory
echo "Files: $(ls | wc -l)"
# Create a file with timestamp
touch "log_$(date +%Y%m%d).txt"
# Use command output in another command
echo "The largest file is: $(ls -S | head -1)"
Tips for Success
- Start with simple patterns and build up to more complex ones
- Use quotes when you want to prevent expansion
- Test your patterns with
echo
before using them in commands - Remember that different shells might handle expansions slightly differently
- Use
set -x
to see how expansions work in real-time
Common Mistakes to Avoid
- Forgetting that spaces in filenames need special handling
- Using the wrong type of quotes (single vs double)
- Not testing patterns before using them with destructive commands
- Forgetting that some expansions need spaces around them
- Mixing up the order of operations in arithmetic expansion
Best Practices
- Use descriptive variable names
- Test expansions with
echo
first - Use quotes when in doubt
- Keep expansions simple and readable
- Comment your code when using complex expansions
Advanced Techniques
Combining Expansions
# Create multiple directories with timestamps
mkdir ~/backups/$(date +%Y%m%d)/{documents,photos,videos}
# Find and process specific files
for file in $(ls *[0-9].txt); do
echo "Processing $file"
done
# Create backup with date
cp important.txt "backup_$(date +%Y%m%d).txt"