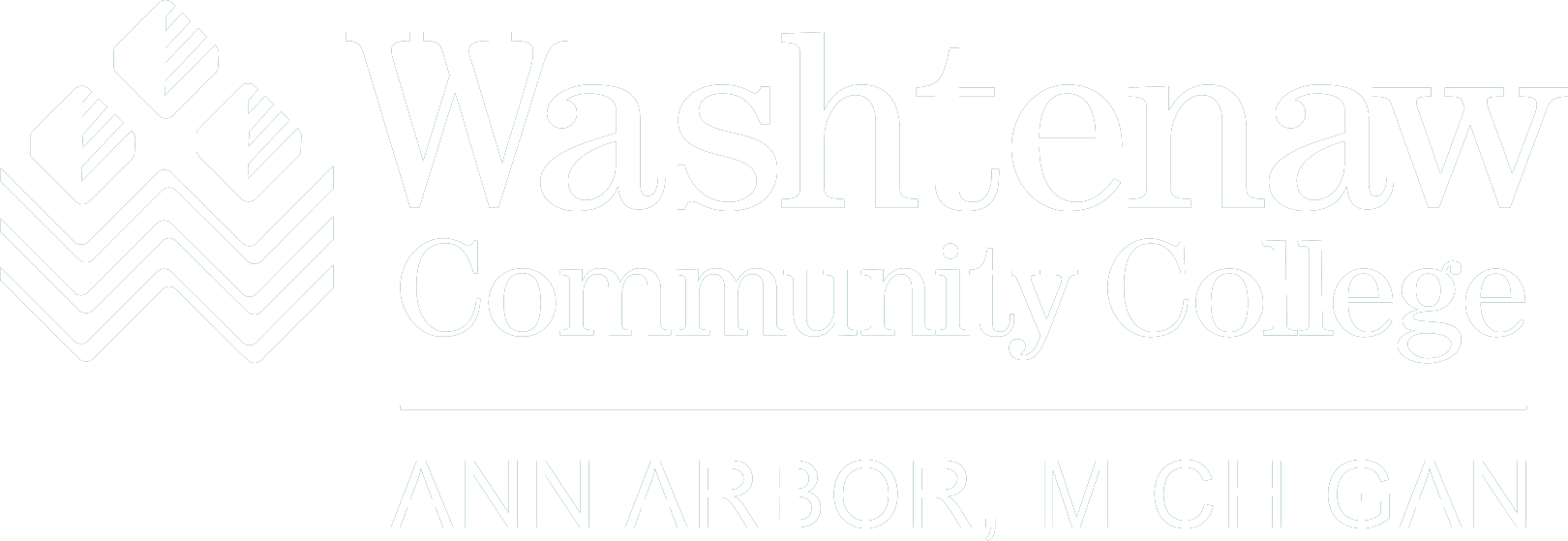
Creating a bash script Comments, Variables and Read
Bash scripts are like mini-programs that can save you hours of work. Think of them as a way to teach your computer to follow instructions - whether it's organizing files, processing data, or performing system maintenance. Once you write a script, you can run it anytime with a single command, and your computer faithfully executes all the steps you've defined.
Let's dive into the world of Bash scripting and discover how easy it is to become the conductor of your computer's orchestra!
Quick Reference: Essential Bash Scripting Elements
Element | Description | Common Use |
---|---|---|
#!/bin/bash |
Shebang line | First line of every script to specify Bash interpreter |
# Comment |
Comments | Adding notes and explanations in your script |
variable="value" |
Variable assignment | Storing data for later use in the script |
echo $variable |
Output text/variables | Displaying information to the user |
read variable |
User input | Getting information from the user |
chmod 700 script.sh |
Change permissions | Making a script executable |
./script.sh |
Run script | Executing a script from its directory |
Creating Your First Bash Script
When to Create a Bash Script
- When you find yourself repeating the same sequence of commands
- When you need to automate system tasks that run regularly
- When you want to create a tool that others can easily use
- When you need to process multiple files in the same way
- When you want to combine multiple commands into a single operation
Common Script Creation Steps
Step | What It Does | When to Use It |
---|---|---|
Add shebang line | Specifies the script interpreter | Always - this should be the first line of every script |
Add comments | Documents what your script does | To explain the purpose of the script and complex commands |
Add commands | The actual work your script performs | These are the same commands you'd type manually |
Save with .sh extension | Makes the file recognizable as a script | When saving your script for the first time |
Make executable | Allows the script to be run directly | After saving and before trying to run the script |
Practical Examples
# Creating your first script
vim hello_world.sh # Open vim editor to create a new file
# Inside the editor, type:
#!/bin/bash
# My first bash script
echo "Hello, World!"
# Save the file, exit vim, then make it executable
chmod 700 hello_world.sh
# Run your script
./hello_world.sh
Understanding and Using Comments
Comments in Bash scripts are lines of text that are ignored by the shell when executing the script. They start with the #
symbol and continue until the end of the line. Comments serve as notes or explanations within your script, making it easier for you and others to understand what the script does and how it works. Think of comments as sticky notes that help you remember why you wrote the code a certain way.
When to Use Comments
- When explaining what a complex command or section does
- When documenting the purpose of the script
- When describing the expected input or output
- When temporarily disabling code without deleting it
- When adding authorship and version information
Common Comment Types
Comment Type | What It Does | When to Use It |
---|---|---|
Header comments | Describes the script's purpose and author | At the beginning of every script |
Inline comments | Explains a specific command on the same line | For complex commands that need explanation |
Section comments | Divides the script into logical sections | In longer scripts to organize code |
Comment blocks | Provides detailed explanation for complex code | Before a group of related commands |
Practical Examples
#!/bin/bash
# Script: backup_files.sh
# Author: Jane Student
# Date: October 2023
# Purpose: Backs up important files to a designated location
# Configuration variables
backupDir="/home/user/backups" # Where files will be backed up
logFile="/home/user/logs/backup.log" # Where we'll log the operation
# Create backup directory if it doesn't exist
mkdir -p $backupDir # -p flag creates parent directories if needed
# Copy important files
cp /home/user/documents/*.docx $backupDir # Copy all Word documents
echo "Backup completed on $(date)" >> $logFile # Log completion time
Working with Variables
Variables in Bash scripts are containers that store data which can be used and manipulated throughout your script. They make scripts more flexible and reusable by allowing you to store values that might change, rather than hardcoding them into the script. A variable name can consist of letters, numbers, and underscores, but cannot start with a number. To assign a value to a variable, use the format variable_name=value
(with no spaces around the equals sign), and to access a variable's value, prefix the variable name with a dollar sign $variable_name
. NOTE: If you try to access a variable that has not been assigned a value, it will return an empty string.
When to Use Variables
- When you need to store data for later use
- When a value will be used multiple times in your script
- When you want to make your script configurable
- When working with user input
- When capturing command output
Common Variable Operations
Operation | What It Does | When to Use It |
---|---|---|
name="value" |
Assigns a value to a variable | When storing data for later use |
echo $name |
Accesses the variable's value | When using the stored data |
${name} |
Explicitly defines variable boundaries | When variable name might be ambiguous |
result=$(command) |
Captures command output | When you need to use command results |
$1, $2, etc. |
Accesses script arguments | When using values passed to the script |
Practical Examples
#!/bin/bash
# Script demonstrating variable usage
# Simple variable assignment
username="student"
courses=3
graduation_year=2025
# Using variables
echo "Hello, $username!"
echo "You are taking $courses courses and will graduate in ${graduation_year}."
# Capturing command output
current_date=$(date +%Y-%m-%d)
echo "Today's date is $current_date"
# Using an environment variable
echo "Your home directory is $HOME"
# Variables with user input
read -p "What is your favorite programming language? " language
echo "$language is a great choice!"
Getting User Input with 'read'
The read
command is Bash's way of accepting input from the user during script execution. When the script encounters a read
statement, it pauses and waits for the user to type something and press Enter. This input is then stored in a variable that you specify, allowing your script to use that information for later processing. The read
command makes your scripts interactive, enabling them to respond differently based on user input rather than following a fixed path.
When to Use the read Command
- When creating interactive scripts
- When you need information from the user to proceed
- When customizing script behavior based on user preferences
- When collecting data for processing
- When requiring authentication or verification
Common read Command Options
Option | What It Does | When to Use It |
---|---|---|
read variable |
Basic input collection | When you need a single value from the user |
read -p "Prompt: " variable |
Shows a prompt before input | When indicating what input is needed |
read -s variable |
Silent input (no display) | When collecting sensitive information like passwords |
read -n 1 variable |
Reads a single character | For yes/no questions or menu selections |
read var1 var2 var3 |
Reads multiple values | When collecting related pieces of information |
Practical Examples
#!/bin/bash
# Script demonstrating the read command
# Basic usage - ask for user's name
echo "What is your name?"
read name
echo "Hello, $name!"
# Using -p for prompt
read -p "What is your age? " age
echo "You are $age years old."
# Reading multiple values
read -p "Enter your first and last name: " first_name last_name
echo "Your name is $first_name $last_name"
# Silent input for passwords
read -sp "Enter your password: " password
echo # Add a newline after password input
echo "Password received (not showing for security)"
# Read with a timeout
read -t 5 -p "Quick! Enter your favorite color (5 seconds): " color
echo "Your favorite color is: $color"
# Single character input
read -n 1 -p "Continue? (y/n): " answer
echo # Add a newline after input
if [ "$answer" = "y" ]; then
echo "Continuing..."
else
echo "Stopping."
fi
Running and Managing Scripts
When to Use Different Running Methods
- When the script is in your current directory
- When the script is in another location
- When you want to test a script without making it executable
- When adding scripts to your system's path
- When scheduling scripts to run automatically
Common Script Execution Methods
Method | What It Does | When to Use It |
---|---|---|
chmod 700 script.sh |
Makes script executable | Before running a script for the first time |
./script.sh |
Runs script from current directory | When the script is in your current location |
bash script.sh |
Explicitly uses bash to run script | When testing or the script isn't executable |
/path/to/script.sh |
Runs script using full path | When the script is in another location |
Add to PATH |
Makes script available everywhere | For frequently used scripts |
Practical Examples
# Making a script executable
chmod 700 myscript.sh # Owner can read, write, execute
# Different ways to run a script
./myscript.sh # Run from current directory
bash myscript.sh # Run with bash explicitly
/home/user/scripts/myscript.sh # Run with full path
# Adding script directory to PATH
echo 'export PATH="$PATH:$HOME/bin"' >> ~/.bashrc
source ~/.bashrc
# Create ~/bin if it doesn't exist
mkdir -p ~/bin
# Move script to bin directory
mv myscript.sh ~/bin/
chmod 700 ~/bin/myscript.sh
# Now you can run it from anywhere
myscript.sh
Learning Aids
Tips for Success
- Start small - Begin with simple scripts that do one thing well, then build up complexity
- Test frequently - Run your script after adding each new feature to catch errors early
- Use meaningful variable names - Choose descriptive names that explain what the variable contains
- Add comments liberally - Your future self will thank you when revisiting old scripts
- Use consistent indentation - Proper spacing makes scripts much easier to read
- Create a scripts directory - Keep all your scripts organized in one place like ~/bin
- Learn from examples - Study other scripts to learn new techniques and best practices
- Use version control - Store your scripts in a Git repository for tracking changes
Common Mistakes to Avoid
- Forgetting spaces around operators - In Bash,
var=5
assigns a value, butvar = 5
tries to run a command called "var" - Misusing quotes - Double quotes allow variable expansion but single quotes don't
- Forgetting the shebang line - Without
#!/bin/bash
, the system might use a different shell to run your script - Not making scripts executable - Forgetting
chmod 700
means you'll get "permission denied" errors - Using spaces in variable names - Variables like
first name
won't work; usefirst_name
orfirstName
instead - Forgetting the $ when using variables - Writing
echo name
prints "name", not the variable's value - Incorrect file paths - Using relative paths can break when running scripts from different locations
- Destructive commands without confirmation - Commands like
rm
should ask for confirmation in scripts
Best Practices
- Use functions for repeated code - Define functions for operations you perform multiple times
- Handle errors gracefully - Check for errors and provide helpful error messages
- Make scripts configurable - Use variables at the top of scripts for easy customization
- Document usage instructions - Include a comment section explaining how to use the script
- Check command success - Verify that commands succeed before proceeding with
if [ $? -eq 0 ]
- Use consistent naming conventions - Adopt a standard naming pattern for all your scripts
- Include script headers - Add author, date, purpose, and usage information at the top
- Validate user input - Never trust user input without checking it first
Summary
Bash scripting is a powerful skill that lets you automate tasks on Linux and Unix-like systems. By creating simple text files with commands, making them executable, and running them, you can save time and reduce errors in repetitive tasks. Variables and user input make your scripts flexible and interactive, while comments ensure they remain understandable over time.
As you continue learning, you'll discover even more powerful features like conditional statements, loops, and functions that will turn your scripts into sophisticated tools. Remember that the best way to learn is by doing - start with simple scripts, gradually add complexity, and before long, you'll be automating tasks you never thought possible!