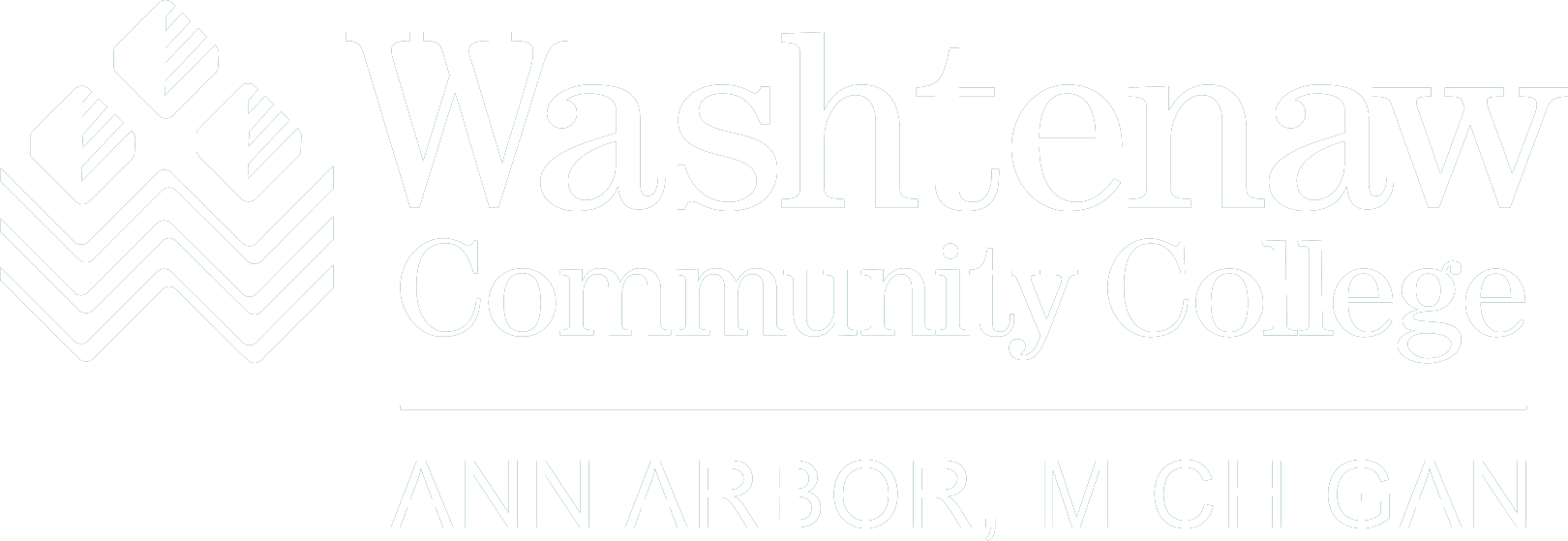
Arrays
Arrays help solve the problem of having to create separate variables for related data. Instead of creating variables like fruit1="apple"
, fruit2="banana"
, and so on, you can simply use one array to keep everything organized and accessible. This makes your scripts cleaner, more efficient, and easier to maintain.
Whether you're managing a list of files to process, storing command results, or working with user inputs, arrays provide a powerful way to keep your data structured and your code elegant. Let's explore how these digital containers can transform the way you handle data in your scripts!
Quick Reference: Array Operations
Operation | Description | Common Use |
---|---|---|
array=("item1" "item2" "item3") |
Array declaration and initialization | Creating a new array with predefined values |
${array[index]} |
Access array element by index | Retrieving a specific element from the array |
array[index]="value" |
Assign value to array element | Updating or adding elements at a specific position |
${array[@]} |
Retrieve all array elements | Processing or displaying the entire array |
${#array[@]} |
Get array length | Determining how many elements are in the array |
array+=(value) |
Append element to array | Adding new elements to the end of an array |
Creating and Using Arrays
When to Use Arrays
- When you need to store multiple related values
- When you want to iterate through a collection of items
- When order of data matters
- When you need to group command outputs or results
- When processing lists of files, users, or any collection of items
Array Operations
Operation | What It Does | When to Use It |
---|---|---|
Creating Arrays | Initializes a new array with values | When starting to work with a collection of data |
Accessing Elements | Retrieves specific items by their position | When you need a particular item from your collection |
Modifying Elements | Changes values at specific positions | When updating information in your collection |
Working with All Elements | Processes entire array at once | When performing bulk operations on your data |
Practical Examples
# Creating an array of fruits
fruits=("apple" "banana" "cherry" "date")
# Accessing a specific element (remember arrays are zero-indexed)
echo "The second fruit is: ${fruits[1]}" # Outputs: banana
# Modifying an element
fruits[3]="dragonfruit" # Replace "date" with "dragonfruit"
echo "Updated fruit list: ${fruits[@]}" # Outputs: apple banana cherry dragonfruit
# Getting the array length
echo "Total number of fruits: ${#fruits[@]}" # Outputs: 4
# Adding a new element to the array
fruits+=("elderberry")
echo "New fruit list: ${fruits[@]}" # Outputs: apple banana cherry dragonfruit elderberry
# Iterating through all elements
for fruit in "${fruits[@]}"
do
echo "I enjoy eating $fruit"
done
# Creating an empty array and adding elements later
vegetables=()
vegetables+=("carrot")
vegetables+=("broccoli")
echo "Vegetable list: ${vegetables[@]}" # Outputs: carrot broccoli
# Creating an array from command output
files=($(ls *.txt)) # Creates an array of all .txt files in current directory
echo "Found ${#files[@]} text files"
Advanced Array Operations
When to Use Advanced Operations
- When you need to manipulate array slices or ranges
- When working with array indexes dynamically
- When you need to remove elements from arrays
- When sorting or processing array elements
- When you want to create associative arrays (key-value pairs)
Advanced Array Techniques
Technique | What It Does | When to Use It |
---|---|---|
Array Slices | Extracts a portion of an array | When you need a subset of your array |
Associative Arrays | Creates arrays with string keys instead of numeric indexes | When you need key-value relationships |
Array Manipulation | Sorting, filtering, and transforming arrays | When processing data collections |
Practical Examples
# Working with array slices
numbers=(1 2 3 4 5 6 7 8 9 10)
echo "Elements 3 through 7: ${numbers[@]:3:5}" # Outputs: 4 5 6 7 8
# Creating an associative array (requires Bash 4+)
declare -A user_info
user_info["name"]="John"
user_info["age"]="25"
user_info["city"]="Boston"
# Accessing elements in associative array
echo "Name: ${user_info["name"]}" # Outputs: John
echo "Age: ${user_info["age"]}" # Outputs: 25
# Iterating through associative array keys and values
for key in "${!user_info[@]}"
do
echo "$key: ${user_info[$key]}"
done
# Removing elements from an array
unset fruits[1] # Removes "banana" but keeps the index
echo "After removal: ${fruits[@]}" # Outputs: apple cherry dragonfruit elderberry
# Re-indexing an array after removal
fruits=("${fruits[@]}") # This re-indexes the array to close the gap
# Sorting an array
sorted_fruits=($(for fruit in "${fruits[@]}"; do echo "$fruit"; done | sort))
echo "Sorted fruits: ${sorted_fruits[@]}"
Learning Aids
Tips for Success
- Always quote array variables - Use
"${array[@]}"
instead of${array[@]}
to preserve spaces in elements - Remember zero-based indexing - The first element is at index 0, not 1
- Check array length before accessing elements - Prevent errors by making sure an index exists
- Use meaningful array names - Name arrays to reflect their contents, like
file_list
oruser_names
- Initialize arrays properly - Use
()
for empty arrays rather than just declaring a variable - Iterate arrays carefully - Use
"${array[@]}"
in for loops to handle elements with spaces - Use associative arrays for complex data - When relationships between data are important
Common Mistakes to Avoid
- Forgetting to quote array references - This can cause issues with spaces in array elements
- Using
${array[*]}
in loops - This doesn't properly separate elements with spaces - Accessing non-existent indexes - Bash silently returns empty strings for non-existent indexes
- Confusing
${#array[@]}
and${#array[0]}
- The first gives array length, the second gives the length of the first element - Not re-indexing after removal -
unset
leaves gaps in indexing that can cause confusion - Using associative arrays without declaring them - Must use
declare -A
before creating associative arrays - Adding elements with
array[index]
out of order - Can create sparse arrays with empty elements
Best Practices
- Use arrays instead of multiple variables - Simplifies code and improves maintainability
- Document array contents - Add comments explaining what each array contains
- Use functions to encapsulate array operations - Makes complex operations reusable and clearer
- Check before accessing - Validate array indexes exist before using them
- Keep arrays focused - Each array should contain a single type of related data
- Be careful with very large arrays - Bash isn't optimized for large data sets
- Consider associative arrays for key-value data - More intuitive than numeric indexes for some data types
Summary
Arrays in Bash provide a powerful way to store, organize, and manipulate collections of related data. Whether you're handling simple lists or complex data structures, arrays help you write cleaner, more efficient code without the need for multiple separate variables.
By mastering array creation, element access, and advanced operations, you'll be able to handle data more effectively in your scripts. Remember to follow best practices like proper quoting and checking array bounds to avoid common pitfalls, and soon arrays will become one of your most valuable tools in the Bash scripting toolkit.